Eliza is a versatile multi-agent simulation framework, built in TypeScript, that allows you to create sophisticated, autonomous AI agents. These agents can interact across multiple platforms while maintaining consistent personalities and knowledge. A key feature that enables this flexibility is the ability to define custom actions and skills. This article will delve into how you can leverage this feature to make your Eliza agents even more powerful.
Understanding Actions in Eliza
Actions are the fundamental building blocks that dictate how Eliza agents respond to and interact with messages. They allow agents to go beyond simple text replies, enabling them to:
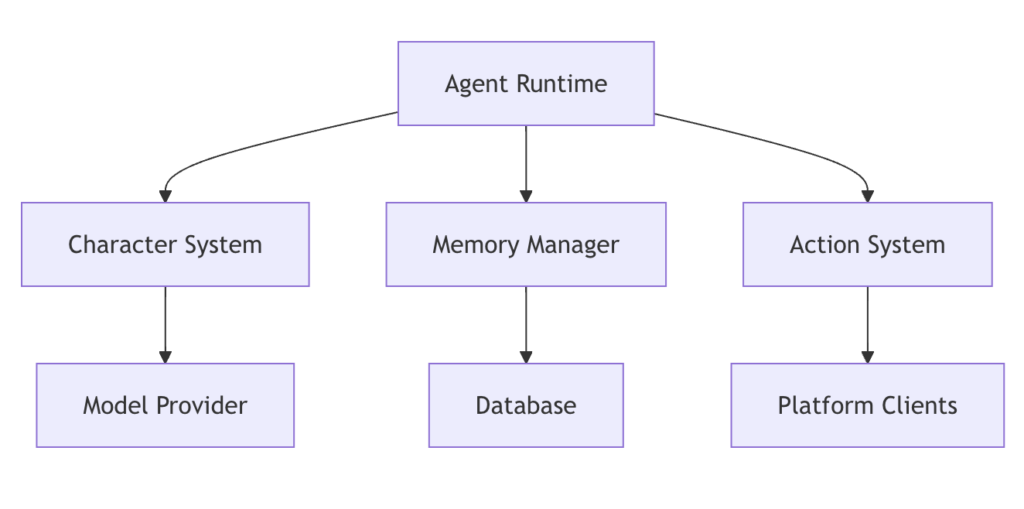
- Interact with external systems.
- Modify their behavior dynamically.
- Perform complex tasks.
Each action in Eliza consists of several key components:
- name: A unique identifier for the action.
- similes: Alternative names or triggers that can invoke the action.
- description: A detailed explanation of what the action does.
- validate: A function that checks if the action is appropriate to execute in the current context.
- handler: The implementation of the action’s behavior – the core logic that the action performs.
- examples: Demonstrates proper usage patterns
- suppressInitialMessage: When set to true, it prevents the initial message from being sent before processing the action.
Built-in Actions
Eliza includes several built-in actions to manage basic conversation flow and external integrations:
- CONTINUE: Keeps a conversation going when more context is required.
- IGNORE: Gracefully disengages from a conversation.
- NONE: Default action for standard conversational replies.
- TAKE_ORDER: Records and processes user purchase orders (primarily for Solana integration).
Creating Custom Actions: Expanding Eliza’s Capabilities
The power of Eliza truly shines when you start implementing custom actions and skills. Here’s how to create them:
- Create a custom_actions directory: This is where you’ll store your action files.
- Add your action files: Each action is defined in its own TypeScript file, implementing the Action interface.
- Configure in elizaConfig.yaml: Point to your custom actions by adding entries under the actions key.
actions:
- name: myCustomAction
path: ./custom_actions/myAction.ts
Action Configuration Structure
Here’s an example of how to structure your action file:
import { Action, IAgentRuntime, Memory } from "@elizaos/core";
export const myAction: Action = {
name: "MY_ACTION",
similes: ["SIMILAR_ACTION", "ALTERNATE_NAME"],
validate: async (runtime: IAgentRuntime, message: Memory) => {
// Validation logic here
return true;
},
description: "A detailed description of your action.",
handler: async (runtime: IAgentRuntime, message: Memory) => {
// The actual logic of your action
return true;
},
};
Implementing a Custom Action
- Validation: Before executing an action, the validate function is called to determine if it can proceed, it checks if all the prerequisites are met to execute a specific action.
- Handler: The handler function contains the core logic of the action. It interacts with the agent runtime and memory and also perform the desired tasks, such as calling external APIs, processing data, or generating output.
Examples of Custom Actions
Here are some examples to illustrate the possibilities:
Basic Action Template:
const customAction: Action = {
name: "CUSTOM_ACTION",
similes: ["SIMILAR_ACTION"],
description: "Action purpose",
validate: async (runtime: IAgentRuntime, message: Memory) => {
// Validation logic
return true;
},
handler: async (runtime: IAgentRuntime, message: Memory) => {
// Implementation
},
examples: [],
};
Advanced Action Example: Processing Documents:
const complexAction: Action = {
name: "PROCESS_DOCUMENT",
similes: ["READ_DOCUMENT", "ANALYZE_DOCUMENT"],
description: "Process and analyze uploaded documents",
validate: async (runtime, message) => {
const hasAttachment = message.content.attachments?.length > 0;
const supportedTypes = ["pdf", "txt", "doc"];
return (
hasAttachment &&
supportedTypes.includes(message.content.attachments[0].type)
);
},
handler: async (runtime, message, state) => {
const attachment = message.content.attachments[0];
// Process document
const content = await runtime
.getService<IDocumentService>(ServiceType.DOCUMENT)
.processDocument(attachment);
// Store in memory
await runtime.documentsManager.createMemory({
id: generateId(),
content: { text: content },
userId: message.userId,
roomId: message.roomId,
});
return true;
},
};
Best Practices for Custom Actions
- Single Responsibility: Ensure each action has a single, well-defined purpose.
- Robust Validation: Always validate inputs and preconditions before executing an action.
- Clear Error Handling: Implement error catching and provide informative error messages.
- Detailed Examples: Include examples in the examples field to show the action’s usage.
Testing Your Actions
Eliza provides a built-in testing framework to validate your actions:
test("Validate action behavior", async () => {
const message: Memory = {
userId: user.id,
content: { text: "Test message" },
roomId,
};
const response = await handleMessage(runtime, message);
// Verify response
});
Custom actions and skills are crucial for unlocking the full potential of Eliza. By creating your own actions, you can tailor Eliza to specific use cases, whether it’s automating complex workflows, integrating with external services, or creating unique, engaging interactions. The flexibility and power provided by this system allow you to push the boundaries of what’s possible with autonomous AI agents.
Reference URLs: